mirror of
https://github.com/yuzu-emu/mbedtls.git
synced 2024-11-23 00:25:39 +01:00
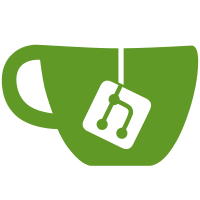
The reported memory leak should have been spotted by make memcheck But it wasn't. Keeping the tests for better coverage.
212 lines
7.3 KiB
Plaintext
212 lines
7.3 KiB
Plaintext
/* BEGIN_HEADER */
|
|
#include "polarssl/rsa.h"
|
|
#include "polarssl/md.h"
|
|
/* END_HEADER */
|
|
|
|
/* BEGIN_DEPENDENCIES
|
|
* depends_on:POLARSSL_PKCS1_V15:POLARSSL_RSA_C:POLARSSL_SHA1_C
|
|
* END_DEPENDENCIES
|
|
*/
|
|
|
|
/* BEGIN_CASE */
|
|
void pkcs1_rsaes_v15_encrypt( int mod, int radix_N, char *input_N, int radix_E,
|
|
char *input_E, int hash,
|
|
char *message_hex_string, char *seed,
|
|
char *result_hex_str, int result )
|
|
{
|
|
unsigned char message_str[1000];
|
|
unsigned char output[1000];
|
|
unsigned char output_str[1000];
|
|
unsigned char rnd_buf[1000];
|
|
rsa_context ctx;
|
|
size_t msg_len;
|
|
rnd_buf_info info;
|
|
|
|
info.length = unhexify( rnd_buf, seed );
|
|
info.buf = rnd_buf;
|
|
|
|
rsa_init( &ctx, RSA_PKCS_V15, hash );
|
|
memset( message_str, 0x00, 1000 );
|
|
memset( output, 0x00, 1000 );
|
|
memset( output_str, 0x00, 1000 );
|
|
|
|
ctx.len = mod / 8 + ( ( mod % 8 ) ? 1 : 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.N, radix_N, input_N ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.E, radix_E, input_E ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_check_pubkey( &ctx ) == 0 );
|
|
|
|
msg_len = unhexify( message_str, message_hex_string );
|
|
|
|
TEST_ASSERT( rsa_pkcs1_encrypt( &ctx, &rnd_buffer_rand, &info, RSA_PUBLIC, msg_len, message_str, output ) == result );
|
|
if( result == 0 )
|
|
{
|
|
hexify( output_str, output, ctx.len );
|
|
|
|
TEST_ASSERT( strcasecmp( (char *) output_str, result_hex_str ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
rsa_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE */
|
|
void pkcs1_rsaes_v15_decrypt( int mod, int radix_P, char *input_P,
|
|
int radix_Q, char *input_Q, int radix_N,
|
|
char *input_N, int radix_E, char *input_E,
|
|
int hash, char *result_hex_str, char *seed,
|
|
char *message_hex_string, int result )
|
|
{
|
|
unsigned char message_str[1000];
|
|
unsigned char output[1000];
|
|
unsigned char output_str[1000];
|
|
rsa_context ctx;
|
|
mpi P1, Q1, H, G;
|
|
size_t output_len;
|
|
rnd_pseudo_info rnd_info;
|
|
((void) seed);
|
|
|
|
mpi_init( &P1 ); mpi_init( &Q1 ); mpi_init( &H ); mpi_init( &G );
|
|
rsa_init( &ctx, RSA_PKCS_V15, hash );
|
|
|
|
memset( message_str, 0x00, 1000 );
|
|
memset( output, 0x00, 1000 );
|
|
memset( output_str, 0x00, 1000 );
|
|
memset( &rnd_info, 0, sizeof( rnd_pseudo_info ) );
|
|
|
|
ctx.len = mod / 8 + ( ( mod % 8 ) ? 1 : 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.P, radix_P, input_P ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.Q, radix_Q, input_Q ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.N, radix_N, input_N ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.E, radix_E, input_E ) == 0 );
|
|
|
|
TEST_ASSERT( mpi_sub_int( &P1, &ctx.P, 1 ) == 0 );
|
|
TEST_ASSERT( mpi_sub_int( &Q1, &ctx.Q, 1 ) == 0 );
|
|
TEST_ASSERT( mpi_mul_mpi( &H, &P1, &Q1 ) == 0 );
|
|
TEST_ASSERT( mpi_gcd( &G, &ctx.E, &H ) == 0 );
|
|
TEST_ASSERT( mpi_inv_mod( &ctx.D , &ctx.E, &H ) == 0 );
|
|
TEST_ASSERT( mpi_mod_mpi( &ctx.DP, &ctx.D, &P1 ) == 0 );
|
|
TEST_ASSERT( mpi_mod_mpi( &ctx.DQ, &ctx.D, &Q1 ) == 0 );
|
|
TEST_ASSERT( mpi_inv_mod( &ctx.QP, &ctx.Q, &ctx.P ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_check_privkey( &ctx ) == 0 );
|
|
|
|
unhexify( message_str, message_hex_string );
|
|
|
|
TEST_ASSERT( rsa_pkcs1_decrypt( &ctx, &rnd_pseudo_rand, &rnd_info, RSA_PRIVATE, &output_len, message_str, output, 1000 ) == result );
|
|
if( result == 0 )
|
|
{
|
|
hexify( output_str, output, ctx.len );
|
|
|
|
TEST_ASSERT( strncasecmp( (char *) output_str, result_hex_str, strlen( result_hex_str ) ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mpi_free( &P1 ); mpi_free( &Q1 ); mpi_free( &H ); mpi_free( &G );
|
|
rsa_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE */
|
|
void pkcs1_rsassa_v15_sign( int mod, int radix_P, char *input_P, int radix_Q,
|
|
char *input_Q, int radix_N, char *input_N,
|
|
int radix_E, char *input_E, int digest, int hash,
|
|
char *message_hex_string, char *salt,
|
|
char *result_hex_str, int result )
|
|
{
|
|
unsigned char message_str[1000];
|
|
unsigned char hash_result[1000];
|
|
unsigned char output[1000];
|
|
unsigned char output_str[1000];
|
|
unsigned char rnd_buf[1000];
|
|
rsa_context ctx;
|
|
mpi P1, Q1, H, G;
|
|
size_t msg_len;
|
|
rnd_buf_info info;
|
|
|
|
info.length = unhexify( rnd_buf, salt );
|
|
info.buf = rnd_buf;
|
|
|
|
mpi_init( &P1 ); mpi_init( &Q1 ); mpi_init( &H ); mpi_init( &G );
|
|
rsa_init( &ctx, RSA_PKCS_V15, hash );
|
|
|
|
memset( message_str, 0x00, 1000 );
|
|
memset( hash_result, 0x00, 1000 );
|
|
memset( output, 0x00, 1000 );
|
|
memset( output_str, 0x00, 1000 );
|
|
|
|
ctx.len = mod / 8 + ( ( mod % 8 ) ? 1 : 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.P, radix_P, input_P ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.Q, radix_Q, input_Q ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.N, radix_N, input_N ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.E, radix_E, input_E ) == 0 );
|
|
|
|
TEST_ASSERT( mpi_sub_int( &P1, &ctx.P, 1 ) == 0 );
|
|
TEST_ASSERT( mpi_sub_int( &Q1, &ctx.Q, 1 ) == 0 );
|
|
TEST_ASSERT( mpi_mul_mpi( &H, &P1, &Q1 ) == 0 );
|
|
TEST_ASSERT( mpi_gcd( &G, &ctx.E, &H ) == 0 );
|
|
TEST_ASSERT( mpi_inv_mod( &ctx.D , &ctx.E, &H ) == 0 );
|
|
TEST_ASSERT( mpi_mod_mpi( &ctx.DP, &ctx.D, &P1 ) == 0 );
|
|
TEST_ASSERT( mpi_mod_mpi( &ctx.DQ, &ctx.D, &Q1 ) == 0 );
|
|
TEST_ASSERT( mpi_inv_mod( &ctx.QP, &ctx.Q, &ctx.P ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_check_privkey( &ctx ) == 0 );
|
|
|
|
msg_len = unhexify( message_str, message_hex_string );
|
|
|
|
if( md_info_from_type( digest ) != NULL )
|
|
TEST_ASSERT( md( md_info_from_type( digest ), message_str, msg_len, hash_result ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_pkcs1_sign( &ctx, &rnd_buffer_rand, &info, RSA_PRIVATE, digest, 0, hash_result, output ) == result );
|
|
if( result == 0 )
|
|
{
|
|
hexify( output_str, output, ctx.len);
|
|
|
|
TEST_ASSERT( strcasecmp( (char *) output_str, result_hex_str ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mpi_free( &P1 ); mpi_free( &Q1 ); mpi_free( &H ); mpi_free( &G );
|
|
rsa_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE */
|
|
void pkcs1_rsassa_v15_verify( int mod, int radix_N, char *input_N, int radix_E,
|
|
char *input_E, int digest, int hash,
|
|
char *message_hex_string, char *salt,
|
|
char *result_hex_str, int result )
|
|
{
|
|
unsigned char message_str[1000];
|
|
unsigned char hash_result[1000];
|
|
unsigned char result_str[1000];
|
|
rsa_context ctx;
|
|
size_t msg_len;
|
|
((void) salt);
|
|
|
|
rsa_init( &ctx, RSA_PKCS_V15, hash );
|
|
memset( message_str, 0x00, 1000 );
|
|
memset( hash_result, 0x00, 1000 );
|
|
memset( result_str, 0x00, 1000 );
|
|
|
|
ctx.len = mod / 8 + ( ( mod % 8 ) ? 1 : 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.N, radix_N, input_N ) == 0 );
|
|
TEST_ASSERT( mpi_read_string( &ctx.E, radix_E, input_E ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_check_pubkey( &ctx ) == 0 );
|
|
|
|
msg_len = unhexify( message_str, message_hex_string );
|
|
unhexify( result_str, result_hex_str );
|
|
|
|
if( md_info_from_type( digest ) != NULL )
|
|
TEST_ASSERT( md( md_info_from_type( digest ), message_str, msg_len, hash_result ) == 0 );
|
|
|
|
TEST_ASSERT( rsa_pkcs1_verify( &ctx, NULL, NULL, RSA_PUBLIC, digest, 0, hash_result, result_str ) == result );
|
|
|
|
exit:
|
|
rsa_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|