mirror of
https://github.com/yuzu-emu/mbedtls.git
synced 2024-11-22 18:05:40 +01:00
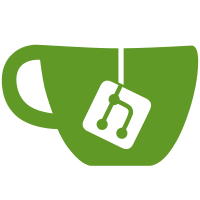
This commit first changes the return convention of EccPoint_mult_safer() so that it properly reports when faults are detected. Then all functions that call it need to be changed to (1) follow the same return convention and (2) properly propagate UECC_FAULT_DETECTED when it occurs. Here's the reverse call graph from EccPoint_mult_safer() to the rest of the library (where return values are translated to the MBEDTLS_ERR_ space) and test functions (where expected return values are asserted explicitly). EccPoint_mult_safer() EccPoint_compute_public_key() uECC_compute_public_key() pkparse.c tests/suites/test_suite_pkparse.function uECC_make_key_with_d() uECC_make_key() ssl_cli.c ssl_srv.c tests/suites/test_suite_pk.function tests/suites/test_suite_tinycrypt.function uECC_shared_secret() ssl_tls.c tests/suites/test_suite_tinycrypt.function uECC_sign_with_k() uECC_sign() pk.c tests/suites/test_suite_tinycrypt.function Note: in uECC_sign_with_k() a test for uECC_vli_isZero(p) is suppressed because it is redundant with a more thorough test (point validity) done at the end of EccPoint_mult_safer(). This redundancy was introduced in a previous commit but not noticed earlier.
111 lines
3.6 KiB
Plaintext
111 lines
3.6 KiB
Plaintext
/* BEGIN_HEADER */
|
|
|
|
#include "tinycrypt/ecc.h"
|
|
#include "tinycrypt/ecc_dh.h"
|
|
#include "tinycrypt/ecc_dsa.h"
|
|
|
|
/* END_HEADER */
|
|
|
|
/* BEGIN_DEPENDENCIES
|
|
* depends_on:MBEDTLS_USE_TINYCRYPT
|
|
* END_DEPENDENCIES
|
|
*/
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_USE_TINYCRYPT */
|
|
void test_ecdh()
|
|
{
|
|
uint8_t private1[NUM_ECC_BYTES] = {0};
|
|
uint8_t private2[NUM_ECC_BYTES] = {0};
|
|
uint8_t public1[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t public2[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t secret1[NUM_ECC_BYTES] = {0};
|
|
uint8_t secret2[NUM_ECC_BYTES] = {0};
|
|
|
|
uECC_set_rng( &uecc_rng_wrapper );
|
|
|
|
TEST_ASSERT( uECC_make_key( public1, private1 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_make_key( public2, private2 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_shared_secret( public2, private1, secret1 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_shared_secret( public1, private2, secret2 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( memcmp( secret1, secret2, sizeof( secret1 ) ) == 0 );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_USE_TINYCRYPT */
|
|
void test_ecdsa()
|
|
{
|
|
uint8_t private[NUM_ECC_BYTES] = {0};
|
|
uint8_t public[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t hash[NUM_ECC_BYTES] = {0};
|
|
uint8_t sig[2*NUM_ECC_BYTES] = {0};
|
|
|
|
uECC_set_rng( &uecc_rng_wrapper );
|
|
|
|
TEST_ASSERT( rnd_std_rand( NULL, hash, NUM_ECC_BYTES ) == 0 );
|
|
|
|
TEST_ASSERT( uECC_make_key( public, private ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_sign( private, hash, sizeof( hash ), sig ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_verify( public, hash, sizeof( hash ), sig ) == UECC_SUCCESS );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_USE_TINYCRYPT */
|
|
void ecdh_primitive_testvec( data_t * private1, data_t * xA_str,
|
|
data_t * yA_str, data_t * private2,
|
|
data_t * xB_str, data_t * yB_str, data_t * z_str )
|
|
{
|
|
uint8_t public1[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t public2[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t secret1[NUM_ECC_BYTES] = {0};
|
|
uint8_t secret2[NUM_ECC_BYTES] = {0};
|
|
|
|
memcpy( public1, xA_str->x, xA_str->len );
|
|
memcpy( public1 + NUM_ECC_BYTES, yA_str->x, yA_str->len );
|
|
memcpy( public2, xB_str->x, xB_str->len );
|
|
memcpy( public2 + NUM_ECC_BYTES, yB_str->x, yB_str->len );
|
|
|
|
// Compute shared secrets and compare to test vector secret
|
|
TEST_ASSERT( uECC_shared_secret( public2, private1->x, secret1 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( uECC_shared_secret( public1, private2->x, secret2 ) == UECC_SUCCESS );
|
|
|
|
TEST_ASSERT( memcmp( secret1, secret2, sizeof( secret1 ) ) == 0 );
|
|
TEST_ASSERT( memcmp( secret1, z_str->x, sizeof( secret1 ) ) == 0 );
|
|
TEST_ASSERT( memcmp( secret2, z_str->x, sizeof( secret2 ) ) == 0 );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_USE_TINYCRYPT */
|
|
void ecdsa_primitive_testvec( data_t * xQ_str, data_t * yQ_str,
|
|
data_t * hash, data_t * r_str, data_t * s_str )
|
|
{
|
|
uint8_t pub_bytes[2*NUM_ECC_BYTES] = {0};
|
|
uint8_t sig_bytes[2*NUM_ECC_BYTES] = {0};
|
|
|
|
memcpy( pub_bytes, xQ_str->x, xQ_str->len );
|
|
memcpy( pub_bytes + NUM_ECC_BYTES, yQ_str->x, yQ_str->len );
|
|
memcpy( sig_bytes, r_str->x, r_str->len );
|
|
memcpy( sig_bytes + NUM_ECC_BYTES, s_str->x, r_str->len );
|
|
|
|
TEST_ASSERT( uECC_verify( pub_bytes, hash->x, hash->len,
|
|
sig_bytes ) == UECC_SUCCESS );
|
|
|
|
// Alter the signature and check the verification fails
|
|
for( int i = 0; i < 2*NUM_ECC_BYTES; i++ )
|
|
{
|
|
uint8_t temp = sig_bytes[i];
|
|
sig_bytes[i] = ( sig_bytes[i] + 1 ) % 256;
|
|
TEST_ASSERT( uECC_verify( pub_bytes, hash->x, hash->len,
|
|
sig_bytes ) == UECC_FAILURE );
|
|
sig_bytes[i] = temp;
|
|
}
|
|
|
|
}
|
|
/* END_CASE */
|