mirror of
https://github.com/yuzu-emu/mbedtls.git
synced 2024-11-23 05:45:49 +01:00
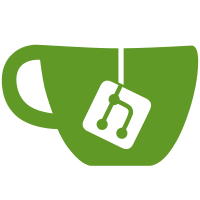
In preparation of changing the type of some parameters of aes_encrypt_ofb() from `char *` to `data_t` to get rid of the calls to mbedtls_test_unhexify(): - Change the name of parameters and local variables to clarify which ones are related to the outputs of the library functions under test and which ones are related to the expected values of those outputs. - Add assertion on fragment_size parameter Signed-off-by: Ronald Cron <ronald.cron@arm.com>
632 lines
24 KiB
Plaintext
632 lines
24 KiB
Plaintext
/* BEGIN_HEADER */
|
|
#include "mbedtls/aes.h"
|
|
/* END_HEADER */
|
|
|
|
/* BEGIN_DEPENDENCIES
|
|
* depends_on:MBEDTLS_AES_C
|
|
* END_DEPENDENCIES
|
|
*/
|
|
|
|
/* BEGIN_CASE */
|
|
void aes_encrypt_ecb( data_t * key_str, data_t * src_str,
|
|
data_t * hex_dst_string, int setkey_result )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
TEST_ASSERT( mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 ) == setkey_result );
|
|
if( setkey_result == 0 )
|
|
{
|
|
TEST_ASSERT( mbedtls_aes_crypt_ecb( &ctx, MBEDTLS_AES_ENCRYPT, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, 16, hex_dst_string->len ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE */
|
|
void aes_decrypt_ecb( data_t * key_str, data_t * src_str,
|
|
data_t * hex_dst_string, int setkey_result )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
TEST_ASSERT( mbedtls_aes_setkey_dec( &ctx, key_str->x, key_str->len * 8 ) == setkey_result );
|
|
if( setkey_result == 0 )
|
|
{
|
|
TEST_ASSERT( mbedtls_aes_crypt_ecb( &ctx, MBEDTLS_AES_DECRYPT, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, 16, hex_dst_string->len ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CBC */
|
|
void aes_encrypt_cbc( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string,
|
|
int cbc_result )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cbc( &ctx, MBEDTLS_AES_ENCRYPT, src_str->len, iv_str->x, src_str->x, output ) == cbc_result );
|
|
if( cbc_result == 0 )
|
|
{
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, src_str->len, hex_dst_string->len ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CBC */
|
|
void aes_decrypt_cbc( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string,
|
|
int cbc_result )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
mbedtls_aes_setkey_dec( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cbc( &ctx, MBEDTLS_AES_DECRYPT, src_str->len, iv_str->x, src_str->x, output ) == cbc_result );
|
|
if( cbc_result == 0)
|
|
{
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, src_str->len, hex_dst_string->len ) == 0 );
|
|
}
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_XTS */
|
|
void aes_encrypt_xts( char *hex_key_string, char *hex_data_unit_string,
|
|
char *hex_src_string, char *hex_dst_string )
|
|
{
|
|
enum { AES_BLOCK_SIZE = 16 };
|
|
unsigned char *data_unit = NULL;
|
|
unsigned char *key = NULL;
|
|
unsigned char *src = NULL;
|
|
unsigned char *dst = NULL;
|
|
unsigned char *output = NULL;
|
|
mbedtls_aes_xts_context ctx;
|
|
size_t key_len, src_len, dst_len, data_unit_len;
|
|
|
|
mbedtls_aes_xts_init( &ctx );
|
|
|
|
data_unit = unhexify_alloc( hex_data_unit_string, &data_unit_len );
|
|
TEST_ASSERT( data_unit_len == AES_BLOCK_SIZE );
|
|
|
|
key = unhexify_alloc( hex_key_string, &key_len );
|
|
TEST_ASSERT( key_len % 2 == 0 );
|
|
|
|
src = unhexify_alloc( hex_src_string, &src_len );
|
|
dst = unhexify_alloc( hex_dst_string, &dst_len );
|
|
TEST_ASSERT( src_len == dst_len );
|
|
|
|
output = zero_alloc( dst_len );
|
|
|
|
TEST_ASSERT( mbedtls_aes_xts_setkey_enc( &ctx, key, key_len * 8 ) == 0 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_xts( &ctx, MBEDTLS_AES_ENCRYPT, src_len,
|
|
data_unit, src, output ) == 0 );
|
|
|
|
TEST_ASSERT( memcmp( output, dst, dst_len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_xts_free( &ctx );
|
|
mbedtls_free( data_unit );
|
|
mbedtls_free( key );
|
|
mbedtls_free( src );
|
|
mbedtls_free( dst );
|
|
mbedtls_free( output );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_XTS */
|
|
void aes_decrypt_xts( char *hex_key_string, char *hex_data_unit_string,
|
|
char *hex_dst_string, char *hex_src_string )
|
|
{
|
|
enum { AES_BLOCK_SIZE = 16 };
|
|
unsigned char *data_unit = NULL;
|
|
unsigned char *key = NULL;
|
|
unsigned char *src = NULL;
|
|
unsigned char *dst = NULL;
|
|
unsigned char *output = NULL;
|
|
mbedtls_aes_xts_context ctx;
|
|
size_t key_len, src_len, dst_len, data_unit_len;
|
|
|
|
mbedtls_aes_xts_init( &ctx );
|
|
|
|
data_unit = unhexify_alloc( hex_data_unit_string, &data_unit_len );
|
|
TEST_ASSERT( data_unit_len == AES_BLOCK_SIZE );
|
|
|
|
key = unhexify_alloc( hex_key_string, &key_len );
|
|
TEST_ASSERT( key_len % 2 == 0 );
|
|
|
|
src = unhexify_alloc( hex_src_string, &src_len );
|
|
dst = unhexify_alloc( hex_dst_string, &dst_len );
|
|
TEST_ASSERT( src_len == dst_len );
|
|
|
|
output = zero_alloc( dst_len );
|
|
|
|
TEST_ASSERT( mbedtls_aes_xts_setkey_dec( &ctx, key, key_len * 8 ) == 0 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_xts( &ctx, MBEDTLS_AES_DECRYPT, src_len,
|
|
data_unit, src, output ) == 0 );
|
|
|
|
TEST_ASSERT( memcmp( output, dst, dst_len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_xts_free( &ctx );
|
|
mbedtls_free( data_unit );
|
|
mbedtls_free( key );
|
|
mbedtls_free( src );
|
|
mbedtls_free( dst );
|
|
mbedtls_free( output );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_XTS */
|
|
void aes_crypt_xts_size( int size, int retval )
|
|
{
|
|
mbedtls_aes_xts_context ctx;
|
|
const unsigned char src[16] = { 0 };
|
|
unsigned char output[16];
|
|
unsigned char data_unit[16];
|
|
size_t length = size;
|
|
|
|
mbedtls_aes_xts_init( &ctx );
|
|
memset( data_unit, 0x00, sizeof( data_unit ) );
|
|
|
|
|
|
/* Valid pointers are passed for builds with MBEDTLS_CHECK_PARAMS, as
|
|
* otherwise we wouldn't get to the size check we're interested in. */
|
|
TEST_ASSERT( mbedtls_aes_crypt_xts( &ctx, MBEDTLS_AES_ENCRYPT, length, data_unit, src, output ) == retval );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_XTS */
|
|
void aes_crypt_xts_keysize( int size, int retval )
|
|
{
|
|
mbedtls_aes_xts_context ctx;
|
|
const unsigned char key[] = { 0x01, 0x02, 0x03, 0x04, 0x05, 0x06 };
|
|
size_t key_len = size;
|
|
|
|
mbedtls_aes_xts_init( &ctx );
|
|
|
|
TEST_ASSERT( mbedtls_aes_xts_setkey_enc( &ctx, key, key_len * 8 ) == retval );
|
|
TEST_ASSERT( mbedtls_aes_xts_setkey_dec( &ctx, key, key_len * 8 ) == retval );
|
|
exit:
|
|
mbedtls_aes_xts_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CFB */
|
|
void aes_encrypt_cfb128( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
size_t iv_offset = 0;
|
|
|
|
memset(output, 0x00, 100);
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
|
|
mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cfb128( &ctx, MBEDTLS_AES_ENCRYPT, 16, &iv_offset, iv_str->x, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, 16, hex_dst_string->len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CFB */
|
|
void aes_decrypt_cfb128( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
size_t iv_offset = 0;
|
|
|
|
memset(output, 0x00, 100);
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
|
|
mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cfb128( &ctx, MBEDTLS_AES_DECRYPT, 16, &iv_offset, iv_str->x, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, 16, hex_dst_string->len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CFB */
|
|
void aes_encrypt_cfb8( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
|
|
mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cfb8( &ctx, MBEDTLS_AES_ENCRYPT, src_str->len, iv_str->x, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, src_str->len, hex_dst_string->len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_CFB */
|
|
void aes_decrypt_cfb8( data_t * key_str, data_t * iv_str,
|
|
data_t * src_str, data_t * hex_dst_string )
|
|
{
|
|
unsigned char output[100];
|
|
mbedtls_aes_context ctx;
|
|
|
|
memset(output, 0x00, 100);
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
|
|
mbedtls_aes_setkey_enc( &ctx, key_str->x, key_str->len * 8 );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cfb8( &ctx, MBEDTLS_AES_DECRYPT, src_str->len, iv_str->x, src_str->x, output ) == 0 );
|
|
|
|
TEST_ASSERT( hexcmp( output, hex_dst_string->x, src_str->len, hex_dst_string->len ) == 0 );
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CIPHER_MODE_OFB */
|
|
void aes_encrypt_ofb( int fragment_size, char *hex_key_string,
|
|
char *hex_iv_string, char *hex_src_string,
|
|
char *hex_expected_output_string )
|
|
{
|
|
unsigned char key_str[32];
|
|
unsigned char iv_str[16];
|
|
unsigned char src_str[64];
|
|
unsigned char output[32];
|
|
unsigned char output_string[65];
|
|
mbedtls_aes_context ctx;
|
|
size_t iv_offset = 0;
|
|
int in_buffer_len;
|
|
unsigned char* src_str_next;
|
|
int key_len;
|
|
|
|
memset( key_str, 0x00, sizeof( key_str ) );
|
|
memset( iv_str, 0x00, sizeof( iv_str ) );
|
|
memset( src_str, 0x00, sizeof( src_str ) );
|
|
memset( output, 0x00, sizeof( output ) );
|
|
memset( output_string, 0x00, sizeof( output_string ) );
|
|
mbedtls_aes_init( &ctx );
|
|
|
|
TEST_ASSERT( (size_t)fragment_size < sizeof( output ) );
|
|
TEST_ASSERT( strlen( hex_key_string ) <= ( 32 * 2 ) );
|
|
TEST_ASSERT( strlen( hex_iv_string ) <= ( 16 * 2 ) );
|
|
TEST_ASSERT( strlen( hex_src_string ) <= ( 64 * 2 ) );
|
|
TEST_ASSERT( strlen( hex_expected_output_string ) <= ( 64 * 2 ) );
|
|
|
|
key_len = mbedtls_test_unhexify( key_str, hex_key_string );
|
|
mbedtls_test_unhexify( iv_str, hex_iv_string );
|
|
in_buffer_len = mbedtls_test_unhexify( src_str, hex_src_string );
|
|
|
|
TEST_ASSERT( mbedtls_aes_setkey_enc( &ctx, key_str, key_len * 8 ) == 0 );
|
|
src_str_next = src_str;
|
|
|
|
while( in_buffer_len > 0 )
|
|
{
|
|
TEST_ASSERT( mbedtls_aes_crypt_ofb( &ctx, fragment_size, &iv_offset,
|
|
iv_str, src_str_next, output ) == 0 );
|
|
|
|
mbedtls_test_hexify( output_string, output, fragment_size );
|
|
TEST_ASSERT( strncmp( (char *) output_string, hex_expected_output_string,
|
|
( 2 * fragment_size ) ) == 0 );
|
|
|
|
in_buffer_len -= fragment_size;
|
|
hex_expected_output_string += ( fragment_size * 2 );
|
|
src_str_next += fragment_size;
|
|
|
|
if( in_buffer_len < fragment_size )
|
|
fragment_size = in_buffer_len;
|
|
}
|
|
|
|
exit:
|
|
mbedtls_aes_free( &ctx );
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_CHECK_PARAMS:!MBEDTLS_PARAM_FAILED_ALT */
|
|
void aes_check_params( )
|
|
{
|
|
mbedtls_aes_context aes_ctx;
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
mbedtls_aes_xts_context xts_ctx;
|
|
#endif
|
|
const unsigned char key[] = { 0x01, 0x02, 0x03, 0x04, 0x05, 0x06 };
|
|
const unsigned char in[16] = { 0 };
|
|
unsigned char out[16];
|
|
size_t size;
|
|
const int valid_mode = MBEDTLS_AES_ENCRYPT;
|
|
const int invalid_mode = 42;
|
|
|
|
TEST_INVALID_PARAM( mbedtls_aes_init( NULL ) );
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
TEST_INVALID_PARAM( mbedtls_aes_xts_init( NULL ) );
|
|
#endif
|
|
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_setkey_enc( NULL, key, 128 ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_setkey_enc( &aes_ctx, NULL, 128 ) );
|
|
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_setkey_dec( NULL, key, 128 ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_setkey_dec( &aes_ctx, NULL, 128 ) );
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_xts_setkey_enc( NULL, key, 128 ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_xts_setkey_enc( &xts_ctx, NULL, 128 ) );
|
|
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_xts_setkey_dec( NULL, key, 128 ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_xts_setkey_dec( &xts_ctx, NULL, 128 ) );
|
|
#endif
|
|
|
|
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ecb( NULL,
|
|
valid_mode, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ecb( &aes_ctx,
|
|
invalid_mode, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ecb( &aes_ctx,
|
|
valid_mode, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ecb( &aes_ctx,
|
|
valid_mode, in, NULL ) );
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_CBC)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cbc( NULL,
|
|
valid_mode, 16,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cbc( &aes_ctx,
|
|
invalid_mode, 16,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cbc( &aes_ctx,
|
|
valid_mode, 16,
|
|
NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cbc( &aes_ctx,
|
|
valid_mode, 16,
|
|
out, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cbc( &aes_ctx,
|
|
valid_mode, 16,
|
|
out, in, NULL ) );
|
|
#endif /* MBEDTLS_CIPHER_MODE_CBC */
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_xts( NULL,
|
|
valid_mode, 16,
|
|
in, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_xts( &xts_ctx,
|
|
invalid_mode, 16,
|
|
in, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_xts( &xts_ctx,
|
|
valid_mode, 16,
|
|
NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_xts( &xts_ctx,
|
|
valid_mode, 16,
|
|
in, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_xts( &xts_ctx,
|
|
valid_mode, 16,
|
|
in, in, NULL ) );
|
|
#endif /* MBEDTLS_CIPHER_MODE_XTS */
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_CFB)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( NULL,
|
|
valid_mode, 16,
|
|
&size, out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( &aes_ctx,
|
|
invalid_mode, 16,
|
|
&size, out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( &aes_ctx,
|
|
valid_mode, 16,
|
|
NULL, out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( &aes_ctx,
|
|
valid_mode, 16,
|
|
&size, NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( &aes_ctx,
|
|
valid_mode, 16,
|
|
&size, out, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb128( &aes_ctx,
|
|
valid_mode, 16,
|
|
&size, out, in, NULL ) );
|
|
|
|
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb8( NULL,
|
|
valid_mode, 16,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb8( &aes_ctx,
|
|
invalid_mode, 16,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb8( &aes_ctx,
|
|
valid_mode, 16,
|
|
NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb8( &aes_ctx,
|
|
valid_mode, 16,
|
|
out, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_cfb8( &aes_ctx,
|
|
valid_mode, 16,
|
|
out, in, NULL ) );
|
|
#endif /* MBEDTLS_CIPHER_MODE_CFB */
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_OFB)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ofb( NULL, 16,
|
|
&size, out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ofb( &aes_ctx, 16,
|
|
NULL, out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ofb( &aes_ctx, 16,
|
|
&size, NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ofb( &aes_ctx, 16,
|
|
&size, out, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ofb( &aes_ctx, 16,
|
|
&size, out, in, NULL ) );
|
|
#endif /* MBEDTLS_CIPHER_MODE_OFB */
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_CTR)
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( NULL, 16, &size, out,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( &aes_ctx, 16, NULL, out,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( &aes_ctx, 16, &size, NULL,
|
|
out, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( &aes_ctx, 16, &size, out,
|
|
NULL, in, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( &aes_ctx, 16, &size, out,
|
|
out, NULL, out ) );
|
|
TEST_INVALID_PARAM_RET( MBEDTLS_ERR_AES_BAD_INPUT_DATA,
|
|
mbedtls_aes_crypt_ctr( &aes_ctx, 16, &size, out,
|
|
out, in, NULL ) );
|
|
#endif /* MBEDTLS_CIPHER_MODE_CTR */
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE */
|
|
void aes_misc_params( )
|
|
{
|
|
#if defined(MBEDTLS_CIPHER_MODE_CBC) || \
|
|
defined(MBEDTLS_CIPHER_MODE_XTS) || \
|
|
defined(MBEDTLS_CIPHER_MODE_CFB) || \
|
|
defined(MBEDTLS_CIPHER_MODE_OFB)
|
|
mbedtls_aes_context aes_ctx;
|
|
const unsigned char in[16] = { 0 };
|
|
unsigned char out[16];
|
|
#endif
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
mbedtls_aes_xts_context xts_ctx;
|
|
#endif
|
|
#if defined(MBEDTLS_CIPHER_MODE_CFB) || \
|
|
defined(MBEDTLS_CIPHER_MODE_OFB)
|
|
size_t size;
|
|
#endif
|
|
|
|
/* These calls accept NULL */
|
|
TEST_VALID_PARAM( mbedtls_aes_free( NULL ) );
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
TEST_VALID_PARAM( mbedtls_aes_xts_free( NULL ) );
|
|
#endif
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_CBC)
|
|
TEST_ASSERT( mbedtls_aes_crypt_cbc( &aes_ctx, MBEDTLS_AES_ENCRYPT,
|
|
15,
|
|
out, in, out )
|
|
== MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH );
|
|
TEST_ASSERT( mbedtls_aes_crypt_cbc( &aes_ctx, MBEDTLS_AES_ENCRYPT,
|
|
17,
|
|
out, in, out )
|
|
== MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH );
|
|
#endif
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_XTS)
|
|
TEST_ASSERT( mbedtls_aes_crypt_xts( &xts_ctx, MBEDTLS_AES_ENCRYPT,
|
|
15,
|
|
in, in, out )
|
|
== MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH );
|
|
TEST_ASSERT( mbedtls_aes_crypt_xts( &xts_ctx, MBEDTLS_AES_ENCRYPT,
|
|
(1 << 24) + 1,
|
|
in, in, out )
|
|
== MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH );
|
|
#endif
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_CFB)
|
|
size = 16;
|
|
TEST_ASSERT( mbedtls_aes_crypt_cfb128( &aes_ctx, MBEDTLS_AES_ENCRYPT, 16,
|
|
&size, out, in, out )
|
|
== MBEDTLS_ERR_AES_BAD_INPUT_DATA );
|
|
#endif
|
|
|
|
#if defined(MBEDTLS_CIPHER_MODE_OFB)
|
|
size = 16;
|
|
TEST_ASSERT( mbedtls_aes_crypt_ofb( &aes_ctx, 16, &size, out, in, out )
|
|
== MBEDTLS_ERR_AES_BAD_INPUT_DATA );
|
|
#endif
|
|
}
|
|
/* END_CASE */
|
|
|
|
/* BEGIN_CASE depends_on:MBEDTLS_SELF_TEST */
|
|
void aes_selftest( )
|
|
{
|
|
TEST_ASSERT( mbedtls_aes_self_test( 1 ) == 0 );
|
|
}
|
|
/* END_CASE */
|