mirror of
https://github.com/yuzu-emu/unicorn.git
synced 2024-10-20 10:38:25 +02:00
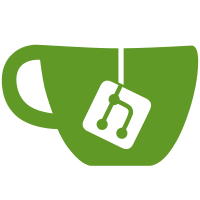
We require a C99 compiler, so let's use 'bool' instead of 'int' when dealing with boolean values. There are few enough clients to fix them all in one pass. Backports commit fc48ffc39ed1060856475e4320d5896f26c945e8 from qemu
69 lines
1.2 KiB
C
69 lines
1.2 KiB
C
/*
|
|
* QBool Module
|
|
*
|
|
* Copyright IBM, Corp. 2009
|
|
*
|
|
* Authors:
|
|
* Anthony Liguori <aliguori@us.ibm.com>
|
|
*
|
|
* This work is licensed under the terms of the GNU LGPL, version 2.1 or later.
|
|
* See the COPYING.LIB file in the top-level directory.
|
|
*
|
|
*/
|
|
|
|
#include "qapi/qmp/qbool.h"
|
|
#include "qapi/qmp/qobject.h"
|
|
#include "qemu-common.h"
|
|
|
|
static void qbool_destroy_obj(QObject *obj);
|
|
|
|
static const QType qbool_type = {
|
|
QTYPE_QBOOL,
|
|
qbool_destroy_obj,
|
|
};
|
|
|
|
/**
|
|
* qbool_from_bool(): Create a new QBool from a bool
|
|
*
|
|
* Return strong reference.
|
|
*/
|
|
QBool *qbool_from_bool(bool value)
|
|
{
|
|
QBool *qb;
|
|
|
|
qb = g_malloc(sizeof(*qb));
|
|
qb->value = value;
|
|
QOBJECT_INIT(qb, &qbool_type);
|
|
|
|
return qb;
|
|
}
|
|
|
|
/**
|
|
* qbool_get_bool(): Get the stored bool
|
|
*/
|
|
bool qbool_get_bool(const QBool *qb)
|
|
{
|
|
return qb->value;
|
|
}
|
|
|
|
/**
|
|
* qobject_to_qbool(): Convert a QObject into a QBool
|
|
*/
|
|
QBool *qobject_to_qbool(const QObject *obj)
|
|
{
|
|
if (qobject_type(obj) != QTYPE_QBOOL)
|
|
return NULL;
|
|
|
|
return container_of(obj, QBool, base);
|
|
}
|
|
|
|
/**
|
|
* qbool_destroy_obj(): Free all memory allocated by a
|
|
* QBool object
|
|
*/
|
|
static void qbool_destroy_obj(QObject *obj)
|
|
{
|
|
assert(obj != NULL);
|
|
g_free(qobject_to_qbool(obj));
|
|
}
|