mirror of
https://github.com/yuzu-emu/unicorn.git
synced 2024-10-20 21:58:18 +02:00
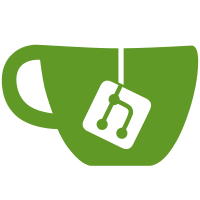
Although accesses to ram_list.dirty_memory[] use atomics so multiple threads can safely dirty the bitmap, the data structure is not fully thread-safe yet. This patch handles the RAM hotplug case where ram_list.dirty_memory[] is grown. ram_list.dirty_memory[] is change from a regular bitmap to an RCU array of pointers to fixed-size bitmap blocks. Threads can continue accessing bitmap blocks while the array is being extended. See the comments in the code for an in-depth explanation of struct DirtyMemoryBlocks. I have tested that live migration with virtio-blk dataplane works. Backports commit 5b82b703b69acc67b78b98a5efc897a3912719eb from qemu
51 lines
1.7 KiB
C
51 lines
1.7 KiB
C
#ifndef RAMLIST_H
|
|
#define RAMLIST_H
|
|
|
|
#include "qemu/queue.h"
|
|
#include "qemu/thread.h"
|
|
|
|
#define DIRTY_MEMORY_CODE 0
|
|
#define DIRTY_MEMORY_NUM 1 /* num of dirty bits */
|
|
|
|
/* The dirty memory bitmap is split into fixed-size blocks to allow growth
|
|
* under RCU. The bitmap for a block can be accessed as follows:
|
|
*
|
|
* rcu_read_lock();
|
|
*
|
|
* DirtyMemoryBlocks *blocks =
|
|
* atomic_rcu_read(&ram_list.dirty_memory[DIRTY_MEMORY_MIGRATION]);
|
|
*
|
|
* ram_addr_t idx = (addr >> TARGET_PAGE_BITS) / DIRTY_MEMORY_BLOCK_SIZE;
|
|
* unsigned long *block = blocks.blocks[idx];
|
|
* ...access block bitmap...
|
|
*
|
|
* rcu_read_unlock();
|
|
*
|
|
* Remember to check for the end of the block when accessing a range of
|
|
* addresses. Move on to the next block if you reach the end.
|
|
*
|
|
* Organization into blocks allows dirty memory to grow (but not shrink) under
|
|
* RCU. When adding new RAMBlocks requires the dirty memory to grow, a new
|
|
* DirtyMemoryBlocks array is allocated with pointers to existing blocks kept
|
|
* the same. Other threads can safely access existing blocks while dirty
|
|
* memory is being grown. When no threads are using the old DirtyMemoryBlocks
|
|
* anymore it is freed by RCU (but the underlying blocks stay because they are
|
|
* pointed to from the new DirtyMemoryBlocks).
|
|
*/
|
|
#define DIRTY_MEMORY_BLOCK_SIZE ((ram_addr_t)256 * 1024 * 8)
|
|
typedef struct {
|
|
//struct rcu_head rcu;
|
|
// Unicorn: unicorn-specific variable to make memory handling less painful
|
|
size_t num_blocks;
|
|
unsigned long *blocks[];
|
|
} DirtyMemoryBlocks;
|
|
|
|
typedef struct RAMList {
|
|
RAMBlock *mru_block;
|
|
QLIST_HEAD(, RAMBlock) blocks;
|
|
DirtyMemoryBlocks *dirty_memory[DIRTY_MEMORY_NUM];
|
|
uint32_t version;
|
|
} RAMList;
|
|
|
|
#endif
|