mirror of
https://github.com/yuzu-emu/unicorn.git
synced 2024-10-21 00:18:22 +02:00
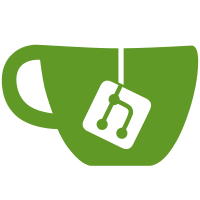
The macro expansions of qdict_put_TYPE() and qlist_append_TYPE() need qbool.h, qnull.h, qnum.h and qstring.h to compile. We include qnull.h and qnum.h in the headers, but not qbool.h and qstring.h. Works, because we include those wherever the macros get used. Open-coding these helpers is of dubious value. Turn them into functions and drop the includes from the headers. This cleanup makes the number of objects depending on qapi/qmp/qnum.h from 4551 (out of 4743) to 46 in my "build everything" tree. For qapi/qmp/qnull.h, the number drops from 4552 to 21. Backports commit 15280c360e54a65e2c7be1a47bfbe41dce1ef986 from qemu
188 lines
3.6 KiB
C
188 lines
3.6 KiB
C
/*
|
|
* QList Module
|
|
*
|
|
* Copyright (C) 2009 Red Hat Inc.
|
|
*
|
|
* Authors:
|
|
* Luiz Capitulino <lcapitulino@redhat.com>
|
|
*
|
|
* This work is licensed under the terms of the GNU LGPL, version 2.1 or later.
|
|
* See the COPYING.LIB file in the top-level directory.
|
|
*/
|
|
|
|
#include "qemu/osdep.h"
|
|
#include "qapi/qmp/qbool.h"
|
|
#include "qapi/qmp/qlist.h"
|
|
#include "qapi/qmp/qnull.h"
|
|
#include "qapi/qmp/qnum.h"
|
|
#include "qapi/qmp/qobject.h"
|
|
#include "qapi/qmp/qstring.h"
|
|
#include "qemu/queue.h"
|
|
#include "qemu-common.h"
|
|
|
|
/**
|
|
* qlist_new(): Create a new QList
|
|
*
|
|
* Return strong reference.
|
|
*/
|
|
QList *qlist_new(void)
|
|
{
|
|
QList *qlist;
|
|
|
|
qlist = g_malloc(sizeof(*qlist));
|
|
qobject_init(QOBJECT(qlist), QTYPE_QLIST);
|
|
QTAILQ_INIT(&qlist->head);
|
|
|
|
return qlist;
|
|
}
|
|
|
|
static void qlist_copy_elem(QObject *obj, void *opaque)
|
|
{
|
|
QList *dst = opaque;
|
|
|
|
qobject_incref(obj);
|
|
qlist_append_obj(dst, obj);
|
|
}
|
|
|
|
QList *qlist_copy(QList *src)
|
|
{
|
|
QList *dst = qlist_new();
|
|
|
|
qlist_iter(src, qlist_copy_elem, dst);
|
|
|
|
return dst;
|
|
}
|
|
|
|
/**
|
|
* qlist_append_obj(): Append an QObject into QList
|
|
*
|
|
* NOTE: ownership of 'value' is transferred to the QList
|
|
*/
|
|
void qlist_append_obj(QList *qlist, QObject *value)
|
|
{
|
|
QListEntry *entry;
|
|
|
|
entry = g_malloc(sizeof(*entry));
|
|
entry->value = value;
|
|
|
|
QTAILQ_INSERT_TAIL(&qlist->head, entry, next);
|
|
}
|
|
|
|
void qlist_append_int(QList *qlist, int64_t value)
|
|
{
|
|
qlist_append(qlist, qnum_from_int(value));
|
|
}
|
|
|
|
void qlist_append_bool(QList *qlist, bool value)
|
|
{
|
|
qlist_append(qlist, qbool_from_bool(value));
|
|
}
|
|
|
|
void qlist_append_str(QList *qlist, const char *value)
|
|
{
|
|
qlist_append(qlist, qstring_from_str(value));
|
|
}
|
|
|
|
void qlist_append_null(QList *qlist)
|
|
{
|
|
qlist_append(qlist, qnull());
|
|
}
|
|
|
|
/**
|
|
* qlist_iter(): Iterate over all the list's stored values.
|
|
*
|
|
* This function allows the user to provide an iterator, which will be
|
|
* called for each stored value in the list.
|
|
*/
|
|
void qlist_iter(const QList *qlist,
|
|
void (*iter)(QObject *obj, void *opaque), void *opaque)
|
|
{
|
|
QListEntry *entry;
|
|
|
|
QTAILQ_FOREACH(entry, &qlist->head, next)
|
|
iter(entry->value, opaque);
|
|
}
|
|
|
|
QObject *qlist_pop(QList *qlist)
|
|
{
|
|
QListEntry *entry;
|
|
QObject *ret;
|
|
|
|
if (qlist == NULL || QTAILQ_EMPTY(&qlist->head)) {
|
|
return NULL;
|
|
}
|
|
|
|
entry = QTAILQ_FIRST(&qlist->head);
|
|
QTAILQ_REMOVE(&qlist->head, entry, next);
|
|
|
|
ret = entry->value;
|
|
g_free(entry);
|
|
|
|
return ret;
|
|
}
|
|
|
|
QObject *qlist_peek(QList *qlist)
|
|
{
|
|
QListEntry *entry;
|
|
QObject *ret;
|
|
|
|
if (qlist == NULL || QTAILQ_EMPTY(&qlist->head)) {
|
|
return NULL;
|
|
}
|
|
|
|
entry = QTAILQ_FIRST(&qlist->head);
|
|
|
|
ret = entry->value;
|
|
|
|
return ret;
|
|
}
|
|
|
|
int qlist_empty(const QList *qlist)
|
|
{
|
|
return QTAILQ_EMPTY(&qlist->head);
|
|
}
|
|
|
|
static void qlist_size_iter(QObject *obj, void *opaque)
|
|
{
|
|
size_t *count = opaque;
|
|
(*count)++;
|
|
}
|
|
|
|
size_t qlist_size(const QList *qlist)
|
|
{
|
|
size_t count = 0;
|
|
qlist_iter(qlist, qlist_size_iter, &count);
|
|
return count;
|
|
}
|
|
|
|
/**
|
|
* qobject_to_qlist(): Convert a QObject into a QList
|
|
*/
|
|
QList *qobject_to_qlist(const QObject *obj)
|
|
{
|
|
if (!obj || qobject_type(obj) != QTYPE_QLIST) {
|
|
return NULL;
|
|
}
|
|
return container_of(obj, QList, base);
|
|
}
|
|
|
|
/**
|
|
* qlist_destroy_obj(): Free all the memory allocated by a QList
|
|
*/
|
|
void qlist_destroy_obj(QObject *obj)
|
|
{
|
|
QList *qlist;
|
|
QListEntry *entry, *next_entry;
|
|
|
|
assert(obj != NULL);
|
|
qlist = qobject_to_qlist(obj);
|
|
|
|
QTAILQ_FOREACH_SAFE(entry, &qlist->head, next, next_entry) {
|
|
QTAILQ_REMOVE(&qlist->head, entry, next);
|
|
qobject_decref(entry->value);
|
|
g_free(entry);
|
|
}
|
|
|
|
g_free(qlist);
|
|
}
|