mirror of
https://github.com/yuzu-emu/unicorn.git
synced 2024-10-20 00:38:21 +02:00
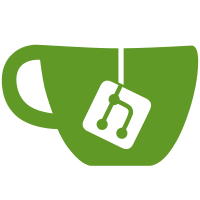
Clean up includes so that osdep.h is included first and headers which it implies are not included manually. This commit was created with scripts/clean-includes. Backports commit aafd758410015e08b1aa8964d739ba8587ce58dc from qemu
82 lines
1.8 KiB
C
82 lines
1.8 KiB
C
/*
|
|
* Wrappers around mutex/cond/thread functions
|
|
*
|
|
* Copyright Red Hat, Inc. 2009
|
|
*
|
|
* Author:
|
|
* Marcelo Tosatti <mtosatti@redhat.com>
|
|
*
|
|
* This work is licensed under the terms of the GNU GPL, version 2 or later.
|
|
* See the COPYING file in the top-level directory.
|
|
*
|
|
*/
|
|
|
|
#include "qemu/osdep.h"
|
|
#include "unicorn/platform.h"
|
|
#ifdef __linux__
|
|
#include <sys/syscall.h>
|
|
#include <linux/futex.h>
|
|
#endif
|
|
#include "qemu/thread.h"
|
|
#include "qemu/atomic.h"
|
|
|
|
static void error_exit(int err, const char *msg)
|
|
{
|
|
fprintf(stderr, "qemu: %s: %s\n", msg, strerror(err));
|
|
abort();
|
|
}
|
|
|
|
int qemu_thread_create(struct uc_struct *uc, QemuThread *thread, const char *name,
|
|
void *(*start_routine)(void*),
|
|
void *arg, int mode)
|
|
{
|
|
sigset_t set, oldset;
|
|
int err;
|
|
pthread_attr_t attr;
|
|
|
|
err = pthread_attr_init(&attr);
|
|
if (err) {
|
|
error_exit(err, __func__);
|
|
return -1;
|
|
}
|
|
if (mode == QEMU_THREAD_DETACHED) {
|
|
err = pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_DETACHED);
|
|
if (err) {
|
|
error_exit(err, __func__);
|
|
return -1;
|
|
}
|
|
}
|
|
|
|
/* Leave signal handling to the iothread. */
|
|
sigfillset(&set);
|
|
pthread_sigmask(SIG_SETMASK, &set, &oldset);
|
|
err = pthread_create(&thread->thread, &attr, start_routine, arg);
|
|
if (err) {
|
|
error_exit(err, __func__);
|
|
return -1;
|
|
}
|
|
|
|
pthread_sigmask(SIG_SETMASK, &oldset, NULL);
|
|
|
|
pthread_attr_destroy(&attr);
|
|
|
|
return 0;
|
|
}
|
|
|
|
void qemu_thread_exit(struct uc_struct *uc, void *retval)
|
|
{
|
|
pthread_exit(retval);
|
|
}
|
|
|
|
void *qemu_thread_join(QemuThread *thread)
|
|
{
|
|
int err;
|
|
void *ret;
|
|
|
|
err = pthread_join(thread->thread, &ret);
|
|
if (err) {
|
|
error_exit(err, __func__);
|
|
}
|
|
return ret;
|
|
}
|