mirror of
https://github.com/yuzu-emu/yuzu.git
synced 2024-10-25 05:15:43 +02:00
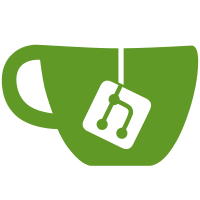
Many of the member variables of the thread class aren't even used outside of the class itself, so there's no need to make those variables public. This change follows in the steps of the previous changes that made other kernel types' members private. The main motivation behind this is that the Thread class will likely change in the future as emulation becomes more accurate, and letting random bits of the emulator access data members of the Thread class directly makes it a pain to shuffle around and/or modify internals. Having all data members public like this also makes it difficult to reason about certain bits of behavior without first verifying what parts of the core actually use them. Everything being public also generally follows the tendency for changes to be introduced in completely different translation units that would otherwise be better introduced as an addition to the Thread class' public interface.
161 lines
5.1 KiB
C++
161 lines
5.1 KiB
C++
// Copyright 2018 yuzu emulator team
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#include <algorithm>
|
|
#include <utility>
|
|
|
|
#include "common/assert.h"
|
|
#include "common/logging/log.h"
|
|
#include "core/arm/arm_interface.h"
|
|
#include "core/core.h"
|
|
#include "core/core_timing.h"
|
|
#include "core/hle/kernel/process.h"
|
|
#include "core/hle/kernel/scheduler.h"
|
|
|
|
namespace Kernel {
|
|
|
|
std::mutex Scheduler::scheduler_mutex;
|
|
|
|
Scheduler::Scheduler(Core::ARM_Interface& cpu_core) : cpu_core(cpu_core) {}
|
|
|
|
Scheduler::~Scheduler() {
|
|
for (auto& thread : thread_list) {
|
|
thread->Stop();
|
|
}
|
|
}
|
|
|
|
bool Scheduler::HaveReadyThreads() const {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
return ready_queue.get_first() != nullptr;
|
|
}
|
|
|
|
Thread* Scheduler::GetCurrentThread() const {
|
|
return current_thread.get();
|
|
}
|
|
|
|
Thread* Scheduler::PopNextReadyThread() {
|
|
Thread* next = nullptr;
|
|
Thread* thread = GetCurrentThread();
|
|
|
|
if (thread && thread->GetStatus() == ThreadStatus::Running) {
|
|
// We have to do better than the current thread.
|
|
// This call returns null when that's not possible.
|
|
next = ready_queue.pop_first_better(thread->GetPriority());
|
|
if (!next) {
|
|
// Otherwise just keep going with the current thread
|
|
next = thread;
|
|
}
|
|
} else {
|
|
next = ready_queue.pop_first();
|
|
}
|
|
|
|
return next;
|
|
}
|
|
|
|
void Scheduler::SwitchContext(Thread* new_thread) {
|
|
Thread* previous_thread = GetCurrentThread();
|
|
|
|
// Save context for previous thread
|
|
if (previous_thread) {
|
|
cpu_core.SaveContext(previous_thread->GetContext());
|
|
// Save the TPIDR_EL0 system register in case it was modified.
|
|
previous_thread->SetTPIDR_EL0(cpu_core.GetTPIDR_EL0());
|
|
|
|
if (previous_thread->GetStatus() == ThreadStatus::Running) {
|
|
// This is only the case when a reschedule is triggered without the current thread
|
|
// yielding execution (i.e. an event triggered, system core time-sliced, etc)
|
|
ready_queue.push_front(previous_thread->GetPriority(), previous_thread);
|
|
previous_thread->SetStatus(ThreadStatus::Ready);
|
|
}
|
|
}
|
|
|
|
// Load context of new thread
|
|
if (new_thread) {
|
|
ASSERT_MSG(new_thread->GetStatus() == ThreadStatus::Ready,
|
|
"Thread must be ready to become running.");
|
|
|
|
// Cancel any outstanding wakeup events for this thread
|
|
new_thread->CancelWakeupTimer();
|
|
|
|
auto previous_process = Core::CurrentProcess();
|
|
|
|
current_thread = new_thread;
|
|
|
|
ready_queue.remove(new_thread->GetPriority(), new_thread);
|
|
new_thread->SetStatus(ThreadStatus::Running);
|
|
|
|
const auto thread_owner_process = current_thread->GetOwnerProcess();
|
|
if (previous_process != thread_owner_process) {
|
|
Core::CurrentProcess() = thread_owner_process;
|
|
SetCurrentPageTable(&Core::CurrentProcess()->VMManager().page_table);
|
|
}
|
|
|
|
cpu_core.LoadContext(new_thread->GetContext());
|
|
cpu_core.SetTlsAddress(new_thread->GetTLSAddress());
|
|
cpu_core.SetTPIDR_EL0(new_thread->GetTPIDR_EL0());
|
|
cpu_core.ClearExclusiveState();
|
|
} else {
|
|
current_thread = nullptr;
|
|
// Note: We do not reset the current process and current page table when idling because
|
|
// technically we haven't changed processes, our threads are just paused.
|
|
}
|
|
}
|
|
|
|
void Scheduler::Reschedule() {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
Thread* cur = GetCurrentThread();
|
|
Thread* next = PopNextReadyThread();
|
|
|
|
if (cur && next) {
|
|
LOG_TRACE(Kernel, "context switch {} -> {}", cur->GetObjectId(), next->GetObjectId());
|
|
} else if (cur) {
|
|
LOG_TRACE(Kernel, "context switch {} -> idle", cur->GetObjectId());
|
|
} else if (next) {
|
|
LOG_TRACE(Kernel, "context switch idle -> {}", next->GetObjectId());
|
|
}
|
|
|
|
SwitchContext(next);
|
|
}
|
|
|
|
void Scheduler::AddThread(SharedPtr<Thread> thread, u32 priority) {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
thread_list.push_back(std::move(thread));
|
|
ready_queue.prepare(priority);
|
|
}
|
|
|
|
void Scheduler::RemoveThread(Thread* thread) {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
thread_list.erase(std::remove(thread_list.begin(), thread_list.end(), thread),
|
|
thread_list.end());
|
|
}
|
|
|
|
void Scheduler::ScheduleThread(Thread* thread, u32 priority) {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
ASSERT(thread->GetStatus() == ThreadStatus::Ready);
|
|
ready_queue.push_back(priority, thread);
|
|
}
|
|
|
|
void Scheduler::UnscheduleThread(Thread* thread, u32 priority) {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
ASSERT(thread->GetStatus() == ThreadStatus::Ready);
|
|
ready_queue.remove(priority, thread);
|
|
}
|
|
|
|
void Scheduler::SetThreadPriority(Thread* thread, u32 priority) {
|
|
std::lock_guard<std::mutex> lock(scheduler_mutex);
|
|
|
|
// If thread was ready, adjust queues
|
|
if (thread->GetStatus() == ThreadStatus::Ready)
|
|
ready_queue.move(thread, thread->GetPriority(), priority);
|
|
else
|
|
ready_queue.prepare(priority);
|
|
}
|
|
|
|
} // namespace Kernel
|